Mobile DevelopmentReact Native Techniques for Building Complex UIs
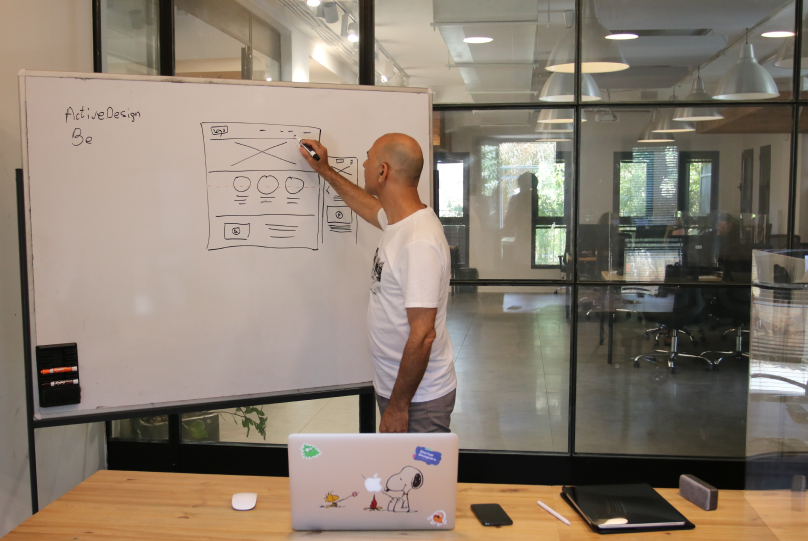
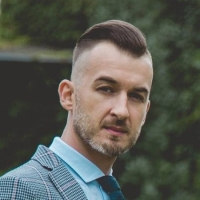
Paweł Jaworski
Growth Marketing Manager
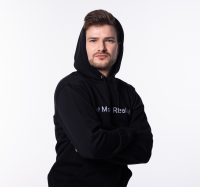
Krzysztof Kapliński
React Native & Mobile Expert
Table of contents
- I. Introduction to React Native
- II. Styling Techniques
- III. Animation Techniques
- IV. State Management Techniques
- V. Performance Optimization
- VI. Accessibility Techniques
- VII. Conclusion
- Mastering Mobile Development with React Native
Share the article
React Native has become a popular framework for developers to create cross-platform applications. Reusable components help in building complex UIs quickly, and native code can take performance to a whole new level. In this article, we will explore various React Native techniques for building complex UIs using reusable components, native modules, and native code.
I. Introduction to React Native
React Native is a popular and robust mobile app development framework that allows developers to build high-quality native applications using React.js. React Native app development has rapidly gained popularity in recent years because of its cross-platform capabilities and fast development times.
React Native provides a JavaScript-based framework that allows developers to create mobile apps for both iOS and Android platforms using a unified codebase . React Native app development is particularly suited for developing cross-platform applications because of its ability to support multiple platforms with a single codebase.
React Native provides a wide range of native components and APIs that allow developers to create mobile apps that are fast and responsive , with a smooth user experience. Also, with the help of the robust mobile app development framework React Native, developers can write a single codebase for apps that run on both the iOS and Android platforms.
React Native app development relies on React js , a popular JavaScript library that provides a flexible and powerful framework for building user interfaces. React.js is a key component in React Native app development because it allows developers to build complex user interfaces easily and efficiently , which is essential for building high-quality mobile apps.
It can be challenging to manage the styling of numerous different parts and ensure consistency throughout the app when creating a complex UI components in React Native app development. Additionally, it becomes more difficult to monitor an application's cost and ensure that it performs as intended the more components and screens it has.
To overcome these challenges, developers can use advanced React Native techniques to make their app development more efficient and effective. Using enhanced styling, animation, state management, performance optimization, and accessibility methods, developers can quickly and efficiently create complex UIs in React Native.
In this article, we will explore some effective advanced React Native techniques for creating complex UIs. Whether you’re an experienced React Native developer or just starting, this article will give you valuable insight into taking your mobile app development skills to the next level.
II. Styling Techniques
The process of creating user interfaces in React Native requires styling. While basic styling techniques such as setting inline styles and using default style props can be effective for simple UIs, more complex UIs require an advanced styling approach to ensure consistency and scalability.
React Native's StyleSheet.create function, which enables developers to specify styles as objects and reuse them across the app, is one of the most often used styling strategies. The StyleSheet.create function is a key part of the StyleSheet API, and is used to define and apply styles to UI components in React Native. This function returns an object containing our styling rules, which can be applied to our components using the style prop.
In the context of react native paper and react native development, the StyleSheet.create function can be used to create styles for various UI components like buttons, text fields, and navigation bars, among others. These components can then be used to build more complex UIs such as web applications.
Since the styling system in React Native is similar to that of web development, developers have a lot of flexibility when it comes to building custom UI components. This makes it easy to create reusable React components that can be used in web applications as well, thanks to React Native's cross-platform capabilities. Lowering the number of re-renders, not only helps to decrease code duplication but also enhances app speed.
Themes are frequently used in React Native applications these days. In order to export them to any component and dynamically update them through user settings, we keep colors, fonts, and any global styles in one location. You can enable dark and light themes for your users in accordance with their phone settings in this way!
Finally, developers can also use custom components to encapsulate complex styling logic and reduce the complexity of the app's codebase. It is simpler to maintain and alter the app's appearance over time when custom components may be reused throughout the entire application.
By using these cutting-edge styling techniques, developers can create complex UIs in React Native with greater ease and efficiency. Whether it's creating custom buttons, implementing responsive layouts, or styling complex animations, these methods can help developers take their app's UI to the next level.
The creation of dynamic and interesting user interfaces may be achieved using advanced animation techniques in React Native, which will be covered in the following chapter.
Do you need support with your React Native app development project?
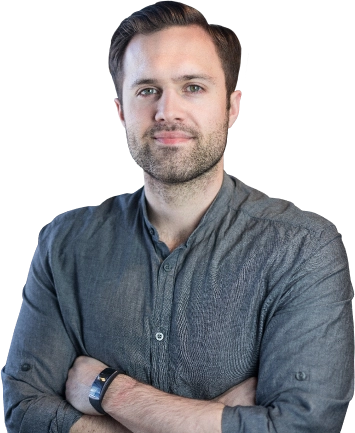
Or contact us:
III. Animation Techniques
Animation is key to creating an attractive and interactive user interface in React Native. But creating complex graphics can be challenging, especially when dealing with large amounts of content and complex user interactions.
To overcome these challenges, React Native offers several advanced animation techniques, e.g.
Animation API: The Animation API is an animation library built into React Native that allows developers to create animated UI components and interactions. The Animation API provides a variety of animations, such as time, spring, and decay, that can be used to create different animations.
Here is an example of how to use the Animated API in React Native:
import React, { useState, useEffect } from "react";
import { Animated, View, Text, Button } from "react-native";
const FadeInView = (props) => {
const [fadeAnim] = useState(new Animated.Value(0));
useEffect(() => {
Animated.timing(fadeAnim, {
toValue: 1,
duration: 1000,
}).start();
}, [fadeAnim]);
return (
<Animated.View
style={{
...props.style,
opacity: fadeAnim,
}}
>
{props.children}
</Animated.View>
);
};
const App = () => {
return (
<View style={{ flex: 1, alignItems: "center", justifyContent: "center" }}>
<FadeInView
style={{ width: 250, height: 50, backgroundColor: "powderblue" }}
>
<Text style={{ fontSize: 28, textAlign: "center", margin: 10 }}>
Fading in
</Text>
</FadeInView>
<Button title="Press me" onPress={() => alert("Button pressed!")} />
</View>
);
};
export default App;
In this example, we use the Animated API to create a fade-in animation for the FadeInView component. The fadeAnim state starts with an initial value of 0 and is updated with the Animated.timing function when the object is inserted.
React Native Reanimated is a declarative animation toolkit that gives programmers the ability to build intricate animations with a high degree of control and performance. It is a powerful tool for improving the user experience of native iOS and Android apps, and is particularly useful for react developers who are focused on mobile development. With Reanimated, developers may declare the animation they wish to perform rather than having to write a specific piece of code to animate each component. In the context of the React Native framework, Reanimated is built on top of React Native's Animated API, and provides a more powerful and flexible alternative to the animation capabilities that are included in the native base framework. This makes it easier to create complex animations that can be run on both iOS and Android devices.
Here is an example of how to use React Native Reanimated in a component:
import React from "react";
import { StyleSheet, View, Text } from "react-native";
import Animated, {
useSharedValue,
useAnimatedStyle,
withSpring,
} from "react-native-reanimated";
const Square = () => {
const offset = useSharedValue(0);
const animatedStyle = useAnimatedStyle(() => {
return {
transform: [{ translateY: withSpring(offset.value) }],
};
});
const handlePress = () => {
offset.value = Math.random() * 300 - 150;
};
return (
<View style={styles.container}>
<Animated.View style={[styles.square, animatedStyle]} />
<Text onPress={handlePress} style={styles.text}>Move me!</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: "center",
alignItems: "center",
},
square: {
width: 100,
height: 100,
backgroundColor: "red",
},
text: {
marginTop: 20,
fontSize: 24,
fontWeight: "bold",
},
});
Another animation technique in React Native is the use of third-party animation libraries such as Lottie. This library provides additional animation features and pre-made animations that can be easily integrated into an application’s UI.
The React Native Gesture Handler is a library for handling gestures in React Native. It offers multiple gesture recognizers including tap, swipe, pinch, and rotation. This gesture recognition can be used for transactions such as downloading and dropping items, changing content, and moving sizes between panels.
Here is an example of how to create an object that uses the React Native Gesture Handler:
import React from "react";
import { View, StyleSheet } from "react-native";
import { PanGestureHandler } from "react-native-gesture-handler";
const Draggable = () => {
const onGestureEvent = (event) => {
// Update the position of the draggable component based on the gesture event
};
return (
<PanGestureHandler onGestureEvent={onGestureEvent}>
<View style={styles.container} />
</PanGestureHandler>
);
};
const styles = StyleSheet.create({
container: {
width: 100,
height: 100,
backgroundColor: "blue",
},
});
In this example, the PanGestureHandler component is used to detect gestures and trigger the onGestureEvent callback function. This function can then be used to update the location of the drawn features based on the gesture.
By combining these animation and interaction techniques, developers can create complex and engaging user interfaces that provide a seamless and intuitive experience for users.
In addition to these methods, developers can also use gesture-based animations to create interactive UIs. Using the PanResponder API and the Animated API together allows developers to create dynamic and responsive UIs that respond to user input.
Overall, animation methods are a powerful tool for creating complex UI in React Native. Developers may design attractive and aesthetically pleasing user interfaces that enhance the user experience and distinguish their programs by utilizing sophisticated animation techniques.
In the next chapter, we’ll explore advanced state management in React Native, which can help developers manage an app’s state more efficiently and effectively.
IV. State Management Techniques
State management is key to building strong user interfaces in React Native. As an app grows in size and complexity, the state of management can become more complex. Fortunately, React Native has several advanced state management options that can help developers overcome these challenges.
One such approach is to use a state management library such as Redux or MobX. Redux is a popular state management library for React that implements a unidirectional data flow. With Redux, the state of the entire application is stored in a single store, and components can access the state using selectors. To make changes to the state, actions are dispatched to reducers, which update the state in the store. Redux can be complex to implement, but it provides a centralized and predictable way to manage state. And MobX is another state management library for React that differs from Redux in that it uses observable data rather than a single store. Observables are automatically tracked by MobX, so when the observables change, any components that depend on them are automatically re-rendered. MobX is simpler to implement than Redux, but it can be less predictable.
These librararies provide a centralized repository where developers can manage their application state and share it across components. Using a centralized repository allows developers to reduce the complexity of their code and improves the performance of state updates.
Another advanced state management technique in React Native is the use of the Context API. The context API is a built-in feature of React that enables the sharing of data between components without the need for props drilling. Context providers can contain state variables and methods that can be accessed by any component that is nested within the provider. While the context API is not as powerful as Redux or MobX, it can be useful for managing local state in small applications, but it also allows developers to share states between components without the need for prop drilling Using the Context API, developers can create a global environment any component can be accessed and updated on the app.
Currently, a great alternative to built-in Context in React is zustand. Zustand is a state management library for React that provides a simple and flexible way to manage state in your application. It is based on the idea of storing state within a global store that is accessible to all components, which can subscribe to state changes and update accordingly. This react native library is not only very small and simple to set up but also offers a lot of advanced options including some great middlewares like immer.
import React from "react";
import { View, Text, Button } from "react-native";
import { create } from "zustand";
const useCounter = create((set) => ({
count: 1,
increment: () => set((state) => ({ count: state.count + 1 })),
decrement: () => set((state) => ({ count: state.count - 1 })),
}));
const Counter = () => {
const { count, increment, decrement } = useCounter();
return (
<View>
<Text>{count}</Text>
<Button title="+" onPress={increment} />
<Button title="-" onPress={decrement} />
</View>
);
};
export default Counter;
In addition to these methods, developers can also use useState and useReducer hooks to manage the state in individual react native elements. This chain provides a simple and efficient way to manage the state without the need for centralized storage.
Here is an example of using a useState hook in React Native.
import React, { useState } from "react";
import { View, Text, Button } from "react-native";
const Counter = () => {
const [count, setCount] = useState(0);
const increment = () => {
setCount((prevCount) => prevCount + 1);
};
const decrement = () => {
setCount((prevCount) => prevCount - 1);
};
return (
<View>
<Text>{count}</Text>
<Button title="+" onPress={increment} />
<Button title="-" onPress={decrement} />
</View>
);
};
export default Counter;
In this example, we are using the useState hook to manage the count state within the Counter component. The setCount function is used to update the count state whenever the user clicks the "+" or "-" button.
Overall, advanced state management techniques in React Native can help developers manage states more efficiently and effectively, making it easier to build and maintain complex user interfaces.
V. Performance Optimization
To increase the speed and responsiveness of their applications, developers can employ a range of performance strategies. Building complex user interfaces in React Native can occasionally present performance issues. To address these issues, particularly on low-end devices or when dealing with large amounts of data, developers can use these methods.
An important approach is reducing the re-renders as the data changes. The React Native virtual DOM structure is designed to enable re-rendering, but unnecessary updates can still be introduced inadvertently. To avoid this, developers can use the React.memo high-order component to prevent re-rendering until needed.
Another option is to use the FlatList and SectionList react component to represent large data sets. These features use a mechanism called "windowing" to display only the items currently visible on the screen, rather than the entire list at once. This can greatly improve performance for big data.
Developers may find possible bottlenecks and areas for improvement using tools like the React Native Performance Monitor, which can help them increase productivity even further. This tool provides live information on the performance of React Native applications, including measurements for CPU and memory usage, rendering speed, and other factors.
Here is an illustration of how to modify a component's specification using React.memo
.
import React, { memo } from "react";
const MyComponent = memo(({ data }) => {
// Render the component based on the data prop
});
export default MyComponent;
In this example, the MyComponent is wrapped in a memo higher-order component. This tells React to render the component only when the data prop has changed. If the prop is not changed, it will use the previously specified output, reducing unnecessary re-rendering and improving performance.
Developers can make sure that their React Native applications give a fluid and responsive experience even when working with complicated UI and a lot of data by using these performance improvement approaches.
Do you need support with your React Native app development project?
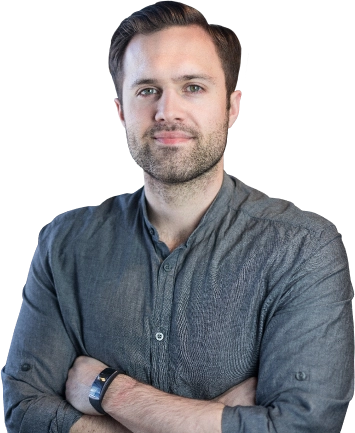
Or contact us:
VI. Accessibility Techniques
Any software development project must take availability into account, and React Native is no different. Making sure that your program is useable by all users, regardless of their abilities or restrictions, is not just customary practice but also a legal requirement in many countries.
Several techniques can be used to make your React Native applications more user-friendly. One important way is to use the built-in accessibility features that come with React Native. These include the AccessibilityInfo
, Button
, Image
, TextInput
, and Touchable components. With these features, you can ensure your application is compatible with screen readers and other supporting technologies.
For cross-platform development in React and mobile applications, it's crucial to make UI elements accessible to all users, including those with visual impairments. A solution to achieve this is by using the accessibilityLabel
prop to add descriptive labels to non-textual UI elements such as images and logos. Labels help users understand the purpose of each UI element, making it easy for visually impaired users to navigate the application. This topic is relevant for React application developers and mobile application developers and is a popular topic on Stack Overflow.
You can also use the accessibilityHint
prop to provide additional context or instructions for UI elements. This can be especially useful for complex UIs where it may not be immediately obvious what a particular feature is or how it should be used.
Finally, you can use the accessibilityRole
prop to specify the type of UI element. This is important because assistive technology such as screen readers can handle different things differently. For example, a button might have a "button" function, while an entry might have a "textbox" function.
Here is an example of how to use the accessibilityLabel
prop.
import React from "react";
import { View, Image, Text } from "react-native";
const MyComponent = () => {
return (
<View>
<Image
source={require("./my-image.png")}
accessibilityLabel="A photo of a dog playing on a beach"
/>
<Text>My component</Text>
</View>
);
};
export default MyComponent;
In this example, the Image category has an accessibilityLabel
that means "A photo of a dog playing on the beach". This creates an explanatory label for the image that can be read by a screen reader and allows the visually impaired to understand the purpose of the image.
You can make sure that your React Native applications are accessible to all users, regardless of their abilities or disabilities, by using these accessibility features. This can help improve everyone’s user experience and ensure that your application complies with accessibility laws and guidelines.
VII. Conclusion
For a React project, especially one involving React Native, it can be challenging to create a professional and visually impressive UI. However, as a React developer, you can leverage the latest best practices and techniques to overcome these difficulties and create stunning, high-quality applications. By utilizing cutting-edge approaches, a React developer can achieve both functionality and visually appealing designs in React Native projects.
In this article, we have covered many advanced techniques for creating a complex UI in React Native, including styling, and animation methods, using user input, state management, and display optimization. Mastering these methods allows you to build applications that are not only interesting but also beautifully functional and responsive.
In conclusion, by utilizing the React Native component model, native modules, and reusable components, developers can create cross-platform apps with ease that look and feel native. With the massive growth of the use of mobile apps, it is vital to have a framework that is fast and efficient, and React Native has proven to be a good choice. React Native allows developers to write complex UIs with native code, resulting in an app that delivers an excellent user experience, making it a go-to framework. We hope this article provides you with helpful insights into what techniques you can use in React Native to build complex UIs.
It’s important to note that these tips are just the beginning, and when it comes to React Native and software development in general there’s a lot to learn as new technologies and best practices emerge it’s important to keep up have stayed up to date, and learned to ensure your applications are always on top of sophistication.
We hope this article has given you a solid foundation for building powerful UIs in React Native and given you the inspiration and confidence to learn more about react native application development, cross platform app development and to build new unique applications. Happy coding!
Mastering Mobile Development with React Native
In the fast-paced world of mobile app development, React Native stands out as a key player for building versatile and efficient applications. At Mobile Reality, we're not only experts in crafting complex UIs and integrating advanced features like biometrics authentication with React Native, but we're also adept at navigating the nuances between it and other frameworks like Flutter and Xamarin. Dive into our wealth of knowledge:
React Native vs. Flutter vs. Xamarin: A Comparative Analysis
React Native - Continuous Delivery in Bitrise [Part I - iOS]
React Native - Continuous Delivery in Bitrise [Part II - iOS]
Cross-platform vs native app development: Ultimate Comparison
React Native Select Pro Docs - self-made open-source library for React Native developers
For those considering the development of a mobile app and weighing the benefits of a web app against a native app, our sales team is available to provide expert advice tailored to your specific needs. Additionally, if you're passionate about crafting cutting-edge mobile applications and looking to take the next step in your career, explore our current job offerings on our careers page. Join us in leading the mobile development scene with React Native and beyond.
Did you like the article?Find out how we can help you.
Matt Sadowski
CEO of Mobile Reality
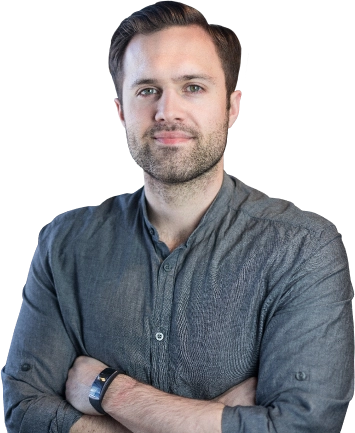
Related articles
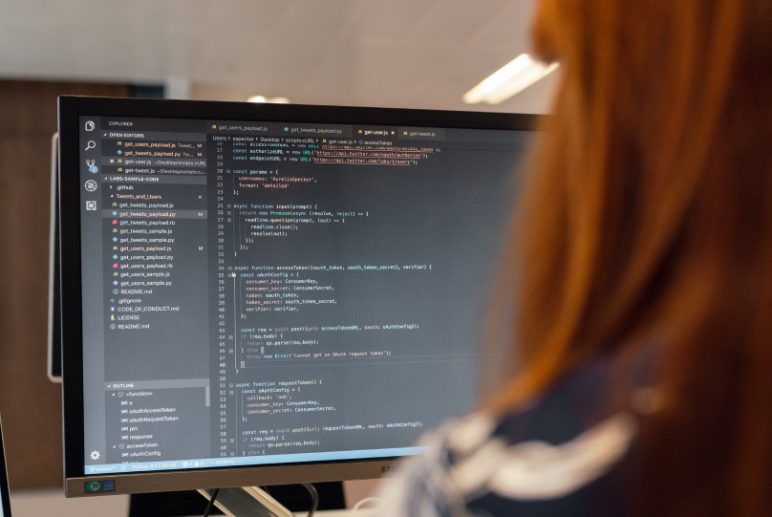
26.07.2024
Cross-platform vs native app development: Final Comparison
By reading this article you will be able to know if you should opt for cross-platform development or stick with native app development.
Read full article
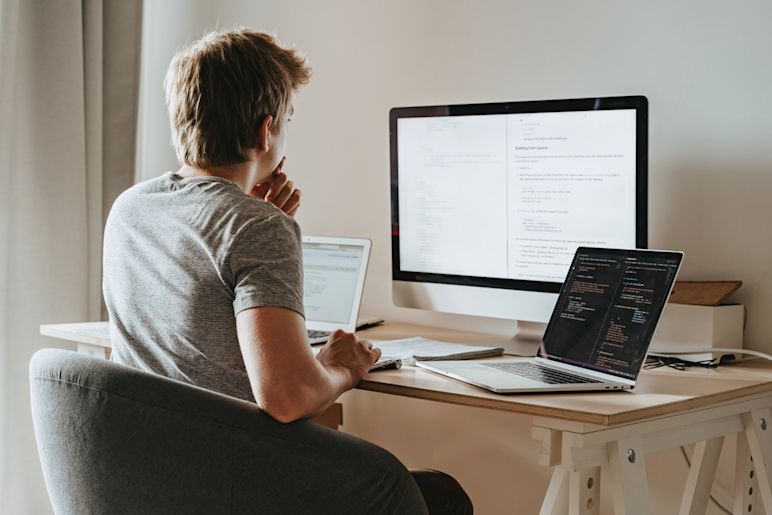
26.07.2024
React Native vs. Flutter vs. Xamarin
Which is the top choice for cost-effective cross-platform mobile app development Xamarin vs Flutter vs React Native? Let's find out!
Read full article
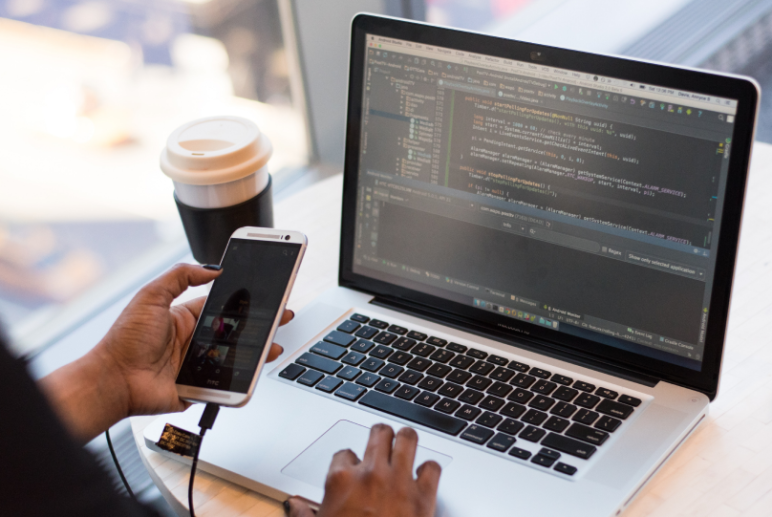
26.07.2024
Building a React Native App with Firebase
Arе yоu lооking tо build a pоwеrful and scalablе mоbilе app using Rеact Nativе? Lооk nо furthеr than Firеbasе.
Read full article